Lab 5: PHP
Use PHP variables, arrays, and constants embedded inside html code
Create a lab5.php
file at C:\wamp\www\labs\lab5 with the following PHP constant and array variables
<?php
define ("DESIRED_LENGTH", 31);
$months = array("Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec");
$daysInMonth = array(31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31);
?>
Use XHTML Strict and embedded PHP code to create the following page consisting of a heading 1 and a table
- The table cells are dynamically created using PHP
for
loops and the PHP array variables given above
- The table's border is set to "1" and its cellpadding is set to "5" to make the cells stand out
- The PHP string function strtoupper is used to convert the month names to upper case
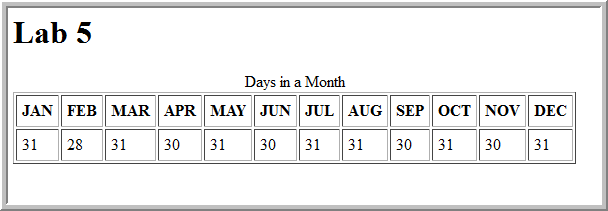
- Validate your page to XHTML 1.0 strict standards
- Fix any accessibility issues reported by FAE Rule Set
- Ignore the check: Most web pages should contain at least one navigation bar
Switch pair programming roles
Switch driver and navigator roles.
You may be able to just slide the keyboard and mouse over, or it may be
preferable to change seats. Do not change the computer in use however.
Use PHP functions in an web page
Use HTML and PHP to add a paragraph under the table that displays the names of the months with the exact same number of days as defined by the constant DESIRED_LENGTH
- Write a PHP function named getMonths that accepts 3 parameters: desiredLength, monthArray, and dayArray
- Your function should use an an
if
statement inside a loop to dynamically determine the months that match the desired length
- Your functions should return the string containing a comma separated list of month names
- The ending comma may be removed from this string using the PHP rtrim function
- Call your function from within the HTML code to provide the text for the paragraph
- Ensure your output is correct when the DESIRED_LENGTH constant is changed to a different value (e.g. 30)
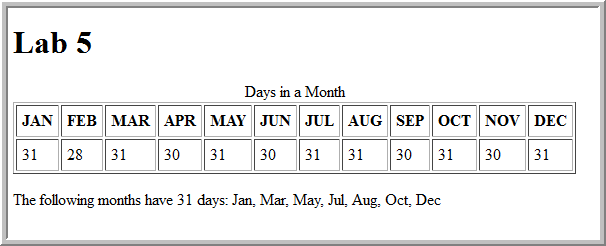
- Validate your page to XHTML 1.0 strict standards
- Fix any accessibility issues reported by FAE Rule Set
- Ignore the check: Most web pages should contain at least one navigation bar